Prototype Pattern
- Create new objects by copying an existing object
Structure
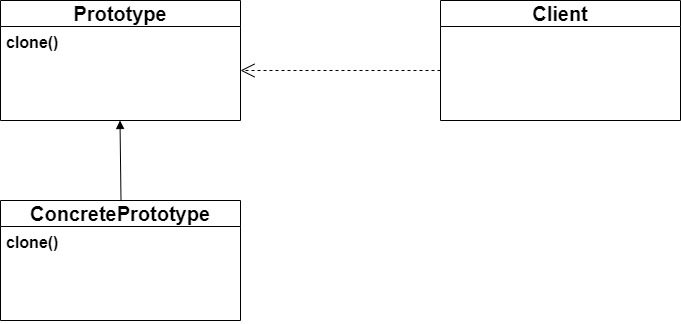
Scenario
- In PowerPoint, add any shape, right click and duplicate
Problem Code
public interface IComponent
{
void Render();
}
public class Circle : IComponent
{
public int Radius { get; set; }
public void Render()
{
Console.WriteLine("Rendering a Circle");
}
}
public class ContextMenu
{
public void Duplicate(IComponent component)
{
if (component is Circle)
{
Circle source = (Circle)component;
Circle target = new Circle();
target.Radius = source.Radius;
//Add target to document
}
}
}
- Violates open closed principle, every time you add new shape, you need to modify the duplicate method
- Coupling between ContextMenu and Circle. ContextMenu needs to know about all shapes and details
Solution
- Move the logic for duplicating the circle from ContextMenu to Circle. Define clone method in circle
- Add clone to Component Interface
- Decouple the ContextMenu from Circle and make it talk to the Component interface
public interface IComponent
{
void Render();
IComponent Clone();
}
public class Circle : IComponent
{
public int Radius { get; set; }
public IComponent Clone()
{
Circle newCircle = new Circle();
newCircle.Radius = Radius;
return newCircle;
}
public void Render()
{
Console.WriteLine("Rendering a Circle");
}
}
public class ContextMenu
{
public void Duplicate(IComponent component)
{
IComponent newComponent = component.Clone();
//Add target to document
}
}
Example Structure
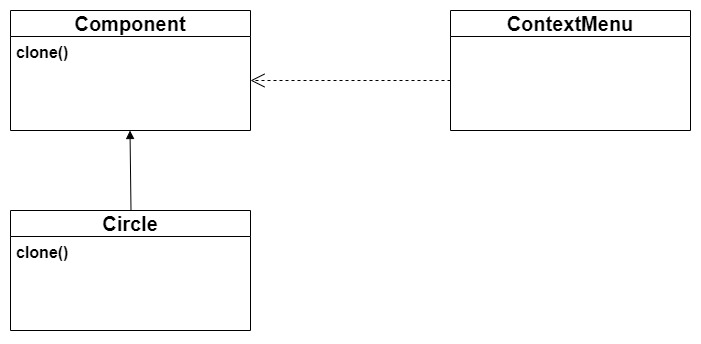
Example Code