Flyweight
- Use this pattern in app where you have large no of objects, and they take significant amount of memory. With flyweight you can reduce the amount of memory consumed by these objects
- Flyweight is an object that we can share
- Separate the data that you need to share, store it in flyweight class and implement factory to cache the objects
Classical Structure
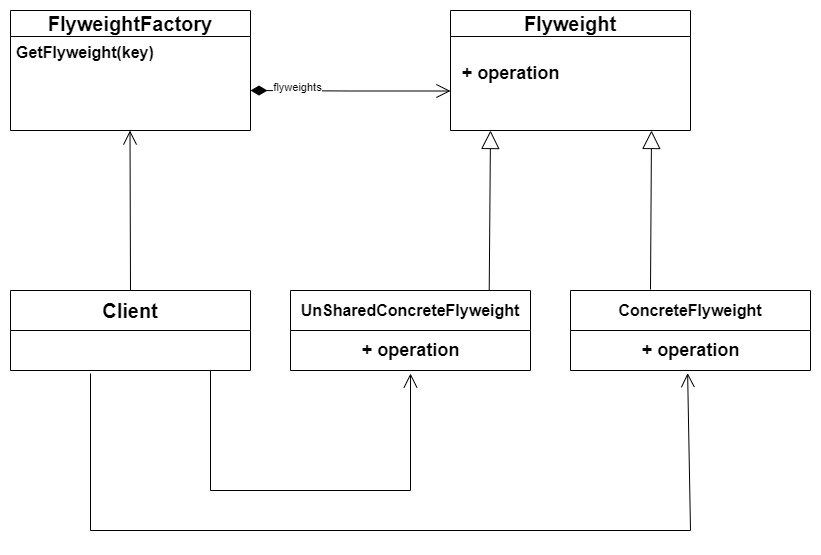
Scenario
- Build a google map mobile app. Render map with various points of interest.
Problem
- If you have 1000 points to render, you need more space
public enum PointType
{
HOSPITAL,
CAFE,
RESTAURANT
}
public class Point
{
private readonly int x;
private readonly int y;
private readonly PointType pointType;
private readonly byte[] icon;
public Point(int x, int y, PointType pointType, byte[] icon)
{
this.x = x;
this.y = y;
this.pointType = pointType;
this.icon = icon;
}
public void Draw()
{
Console.WriteLine($"{pointType} at {x} {y}");
}
}
public class PointService
{
public List<Point> GetPoints()
{
List<Point> points = new List<Point>();
points.Add(new Point(1, 2, PointType.CAFE, null));
return points;
}
}
Solution
public class Point
{
private readonly int x;
private readonly int y;
private readonly PointIcon icon;
public Point(int x, int y, PointIcon icon)
{
this.x = x;
this.y = y;
this.icon = icon;
}
public void Draw()
{
Console.WriteLine($"{icon.PointType} at {x} {y}");
}
}
public class PointIcon
{
public PointType PointType { get; init; }
public byte[] icon { get; init; }
public PointIcon(PointType pointType, byte[] icon)
{
PointType = pointType;
this.icon = icon;
}
}
public class PointIconFactory
{
Dictionary<PointType, PointIcon> icons = new Dictionary<PointType, PointIcon>();
public PointIcon GetPointIcon(PointType pointType)
{
if(!icons.ContainsKey(pointType))
icons.Add(pointType, new PointIcon(pointType, null));
return icons[pointType];
}
}
public class PointService
{
private readonly PointIconFactory pointIconFactory;
public PointService(PointIconFactory pointIconFactory)
{
this.pointIconFactory = pointIconFactory;
}
public List<Point> GetPoints()
{
List<Point> points = new List<Point>();
points.Add(new Point(1, 2, pointIconFactory.GetPointIcon(PointType.CAFE)));
return points;
}
}
Example Structure
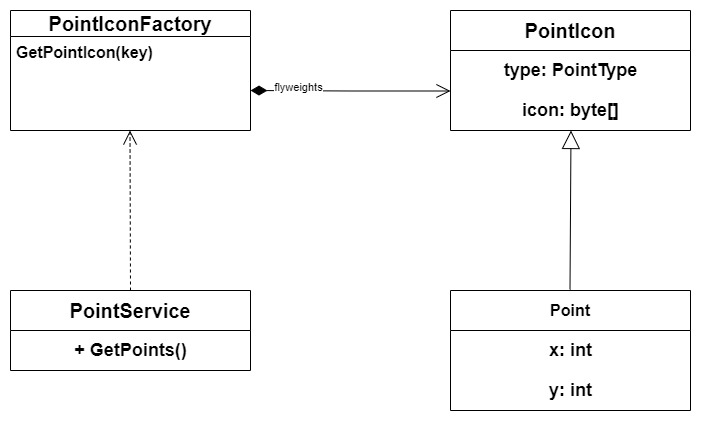
Example Code