Bridge
- Acts as a bridge between 2 independent hierarchies
- Build flexible hierarchies that can grow independent of each other.
Classical Structure
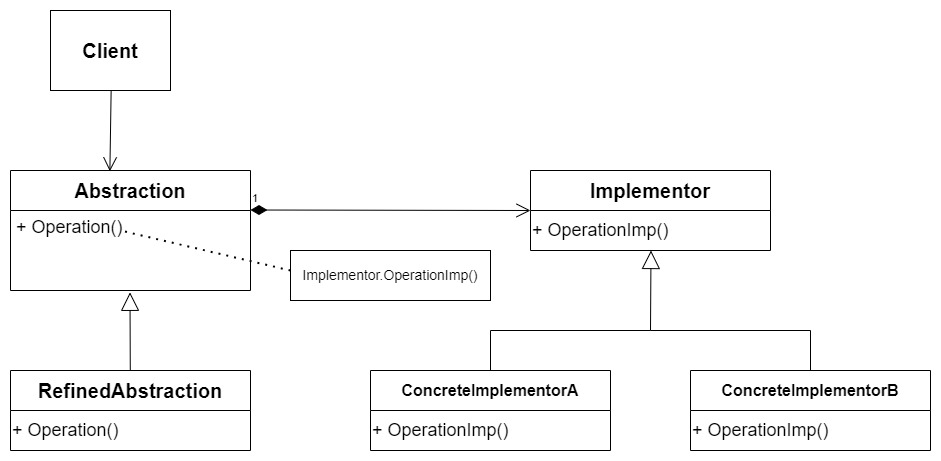
Scenario
- Build a remote control app to control various brands of TV
Problem
public abstract class RemoteControl
{
public abstract void TurnOn();
public abstract void TurnOff();
}
public abstract class AdvancedRemoteControl : RemoteControl
{
public abstract void SetChannel(int number);
}
public class SonyRemoteControl : RemoteControl
{
public override void TurnOff()
{
Console.WriteLine("Turn off");
}
public override void TurnOn()
{
Console.WriteLine("Turn on");
}
}
public class SonyAdvancedRemoteControl : AdvancedRemoteControl
{
public override void SetChannel(int number)
{
Console.WriteLine("Set channel");
}
public override void TurnOff()
{
Console.WriteLine("Turn off");
}
public override void TurnOn()
{
Console.WriteLine("Turn on");
}
}
- Every time you want to support a new TV brand, you have to create concrete classes for that
- Also every time you introduce a new remote control (eg MovieRemoteControl), you have to provide concrete class for all brands
- Hierarchy gets more complex, every time you want to support a new TV brand or new remote control
- Hierarchy grows into 2 different dimensions (feature and implementation)
Solution
- Whenever hierarchy grows into 2 different dimensions, split into 2 separate hierarchies and connect them using bridge
public interface IDevice
{
void TurnOn();
void TurnOff();
void SetChannel(int number);
}
public class SonyTV : IDevice
{
public void SetChannel(int number)
{
Console.WriteLine("SonyTV Set Channel");
}
public void TurnOff()
{
Console.WriteLine("SonyTV Turn Off");
}
public void TurnOn()
{
Console.WriteLine("SonyTV Turn On");
}
}
public class SamsungTV: IDevice
{
public void SetChannel(int number)
{
Console.WriteLine("SamsungTV Set Channel");
}
public void TurnOff()
{
Console.WriteLine("SamsungTV Turn Off");
}
public void TurnOn()
{
Console.WriteLine("SamsungTV Turn On");
}
}
public class RemoteControl
{
protected IDevice device;
public RemoteControl(IDevice device)
{
this.device = device;
}
public void TurnOn()
{
device.TurnOn();
}
public void TurnOff()
{
device.TurnOff();
}
}
public class AdvancedRemoteControl : RemoteControl
{
public AdvancedRemoteControl(IDevice device) : base(device)
{
}
public void SetChannel(int number)
{
device.SetChannel(number);
}
}
public class Program
{
static void Main(string[] args)
{
var remoteControl = new AdvancedRemoteControl(new SamsungTV());
remoteControl.TurnOn();
}
}
Example Structure
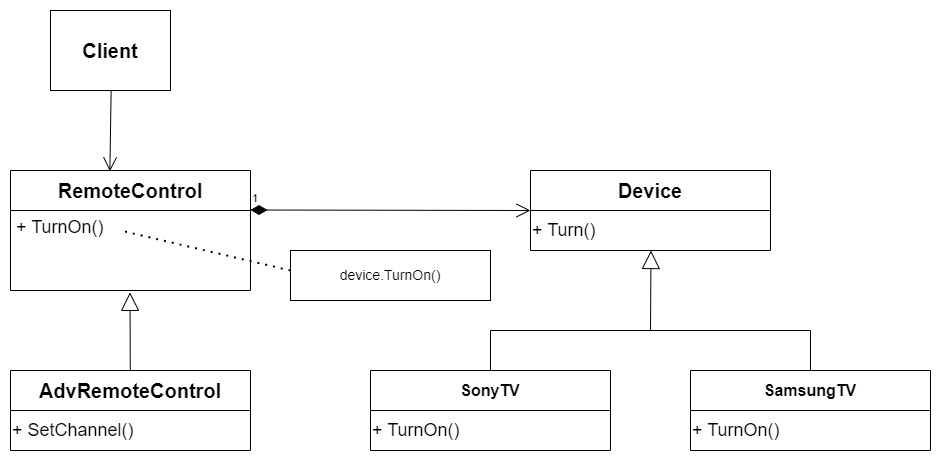
Example Code