Composite Pattern
- Use it to represent a hierarchy of objects and treat the objects in the hierarchy the same way.
Scenario
- Powerpoint with individual object and group of objects
Classical Structure
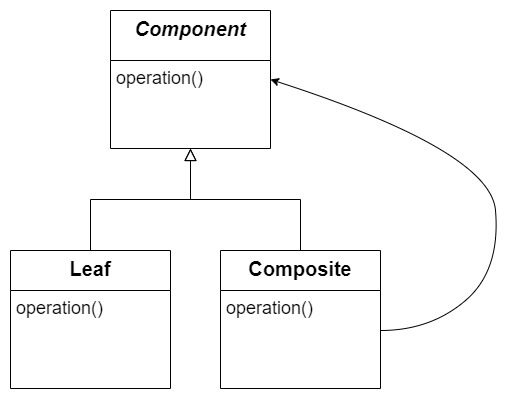
Problem
- Need to check the type of the object and cast it to new type and call method on it
- You need to repeat the same logic (iterating) for all methods
public class Shape
{
public void Render()
{
Console.WriteLine("Rendering Shape");
}
}
public class Group
{
List<Object> objects = new List<object>();
public void Add(Object obj)
{
objects.Add(obj);
}
public void Render()
{
foreach (var item in objects)
{
if (item is Shape)
{
((Shape)item).Render();
}
else
{
((Group)item).Render();
}
}
}
}
Solution
public interface IComponent
{
void Render();
}
public class Shape: IComponent
{
public void Render()
{
Console.WriteLine("Rendering Shape");
}
}
public class Group: IComponent
{
List<IComponent> components = new List<IComponent>();
public void Add(IComponent obj)
{
components.Add(obj);
}
public void Render()
{
foreach (var item in components)
{
item.Render();
}
}
}
public class Program
{
public static void Main(string[] args)
{
var group1 = new Group();
group1.Add(new Shape());
group1.Add(new Shape());
group1.Render();
var group2 = new Group();
group2.Add(new Shape());
group2.Add(new Shape());
group2.Render();
var group = new Group();
group.Add(group1);
group.Add(group2);
}
}
Example Structure
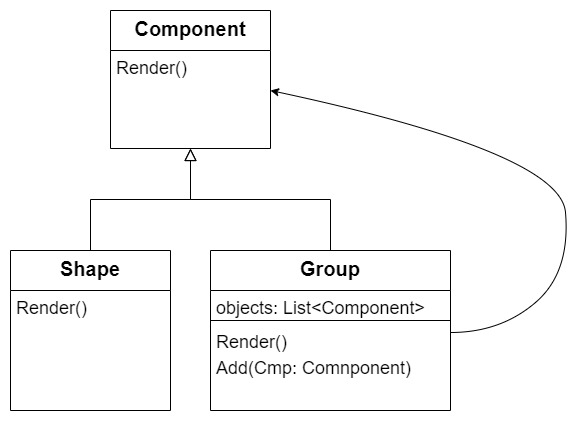
Example Code