Facade (Front)
- Use this pattern to provide a simple interface to a complex system
- Facade reduces coupling
Classical Structure
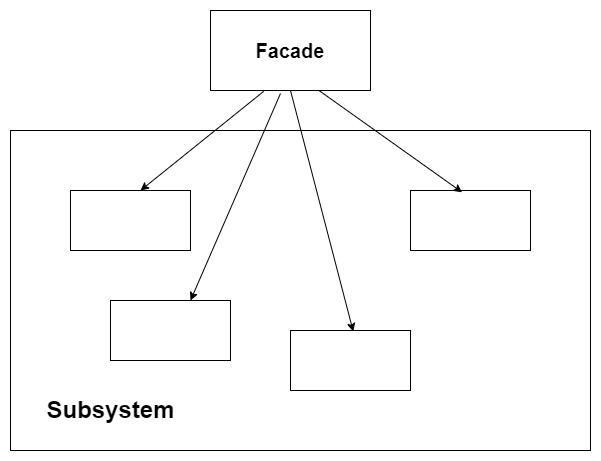
Scenario
- Sending push notifications to users
Problem
- Everytime to send a push notification you have to follow lot of steps.
- Main class is coupled or dependent on these classes (AuthToken, Connection, Message, NotificationServer) for sending notification
- If other classes need to send notification, they also are coupled to these classes
- If any of these classes change several classes might be affected.
public class Connection
{
public void Disconnect()
{
}
}
public class AuthToken
{
}
public class Message
{
private readonly string content;
public Message(string content)
{
this.content = content;
}
}
public class NotificationServer
{
public Connection Connect(string ipAddress)
{
return new Connection();
}
public AuthToken Authenticate(string appID, string key)
{
return new AuthToken();
}
public void Send(AuthToken token, Message message, string target)
{
Console.WriteLine("Sending a message");
}
}
public class Program
{
public static void Main()
{
var server = new NotificationServer();
var connection = server.Connect("ip");
var authToken = server.Authenticate("AppId", "key");
var message = new Message("Hello World");
server.Send(authToken, message, "target");
connection.Disconnect();
}
}
Solution
- Encapsulates all logic to send push notification
- Hide all complexity behind facade
public class NotificationService
{
public void Send(string message, string target)
{
var server = new NotificationServer();
var connection = server.Connect("ip");
var authToken = server.Authenticate("AppId", "key");
server.Send(authToken, new Message(message), target);
connection.Disconnect();
}
}
public class Program
{
public static void Main()
{
var service = new NotificationService();
service.Send("Hello World", "target");
}
}
Example Code