Command
- Turns/Encapsulates a request into a stand-alone object that contains all information about the request. This transformation lets you pass requests as a method argument, delay or queue a request’s execution, and support undoable operations.
- Used in lot of frameworks
Classical Structure
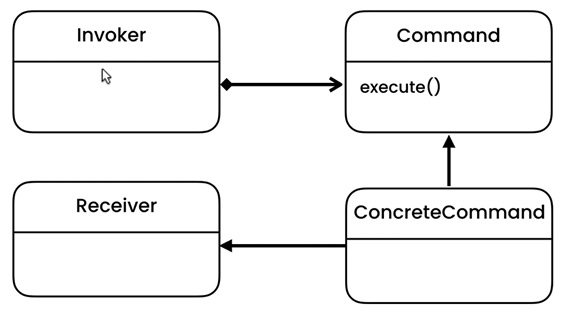
Scenario
- Build a GUI framework to build app with it. In the framework define classes like Button, TextBox, CheckBox, so developers can use it to build forms
Solution
- Decoupling the Sender/Invoker from the Receiver
- You can keep track of all commands that are executed. Repla, undo
public interface ICommand
{
void Execute();
}
public class Button
{
private readonly ICommand command;
public Button(ICommand command)
{
this.command = command;
}
public void Click()
{
command.Execute();
}
}
public class CustomerService
{
public void AddCustomer()
{
Console.WriteLine("Add customer");
}
}
public class AddCustomerCommand : ICommand
{
CustomerService customerService;
public AddCustomerCommand(CustomerService customerService)
{
this.customerService = customerService;
}
public void Execute()
{
customerService.AddCustomer();
}
}
Example Structure
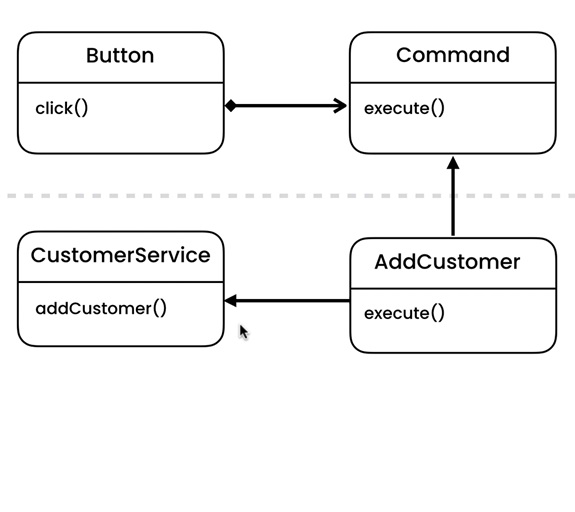
Example Code
Editor Example