Strategy
- Defines a family of algorithms, encapsulate each one, and make them interchangeable.
Classical Structure
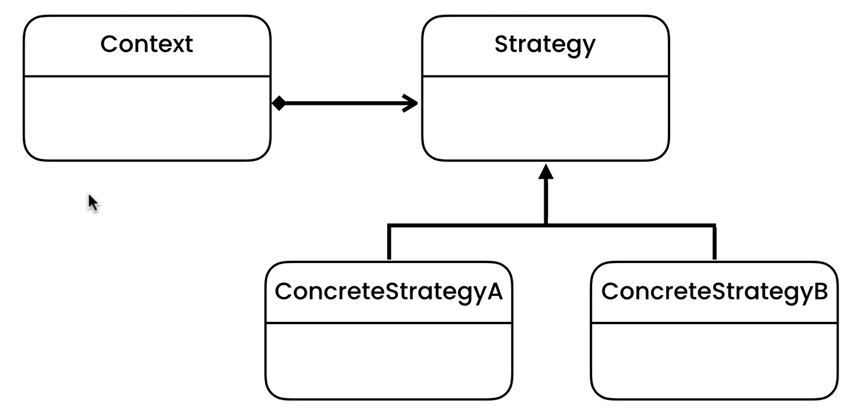
Scenario
- Build a class for storing image after compressing and applying filter
Problem
- Violates SRP
- Violates OCP
public class ImageStorage
{
private string compressor;
private string filter;
public ImageStorage(string compressor, string filter)
{
this.compressor = compressor;
this.filter = filter;
}
public void Store()
{
if(compressor == "JPG")
Console.WriteLine("Compressing image using JPG");
else if(compressor == "PNG")
Console.WriteLine("Compressing image using PNG");
if (filter == "BW")
Console.WriteLine("Apply filter BW");
else if (filter == "HC")
Console.WriteLine("Apply high contrast");
}
}
Solution
public interface ICompressor
{
void Compress(string fileName);
}
public class JPGCompressor : ICompressor
{
public void Compress(string fileName)
{
Console.WriteLine("Compressing image");
}
}
public interface IFilter
{
void Apply(string fileName);
}
public class BlackAndWhiteFilter : IFilter
{
public void Apply(string fileName)
{
Console.WriteLine("Applying BW filter");
}
}
public class ImageStorage
{
private ICompressor compressor;
private IFilter filter;
public ImageStorage(ICompressor compressor, IFilter filter)
{
this.compressor = compressor;
this.filter = filter;
}
public void Store(string fileName)
{
compressor.Compress(fileName);
filter.Apply(fileName);
}
}
public class Program
{
static void Main(string[] args)
{
var imageStorage = new ImageStorage(new JPGCompressor(), new BlackAndWhiteFilter());
imageStorage.Store("a");
}
}
Example Structure
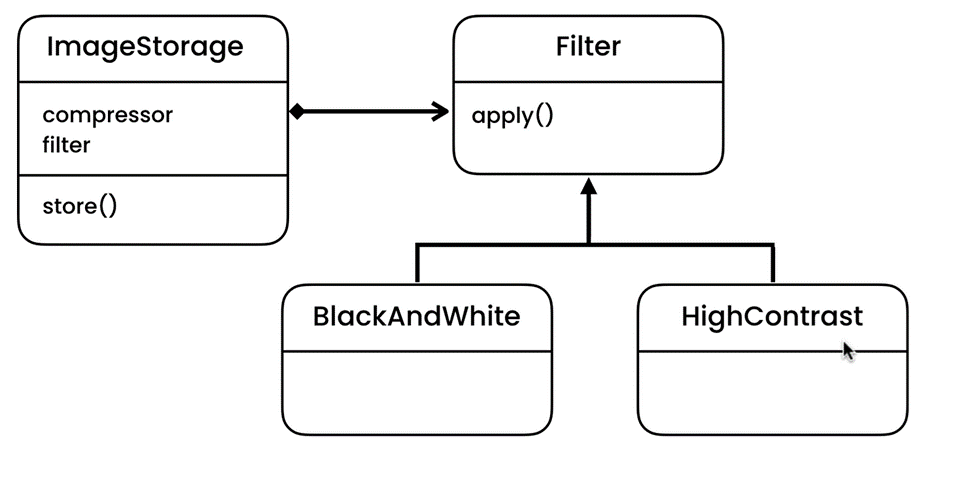
Example Code