Adapter Pattern
- You use this pattern to convert the interface of class to a different form that matches what we need
Classical Structure
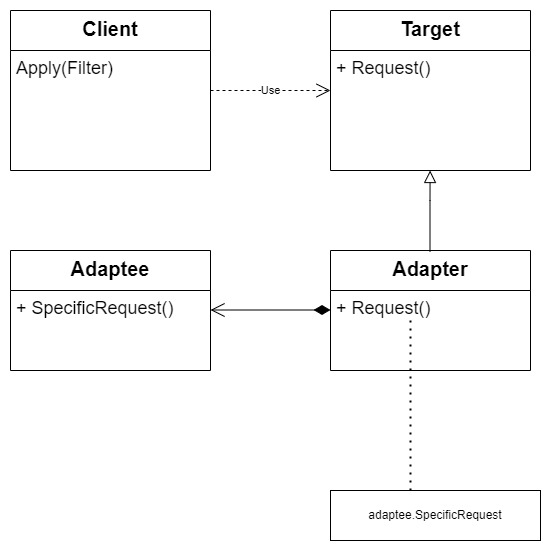
Scenario
Problem
- Interface of caramel class doesn't match what apply method of ImageView expects because Apply method expects class that implements IFilter.
public class Image
{
}
public interface IFilter
{
void Apply(Image image);
}
public class VividFilter : IFilter
{
public void Apply(Image image)
{
Console.WriteLine("Applying vivid filter");
}
}
public class ImageView
{
private readonly Image image;
public ImageView(Image image)
{
this.image = image;
}
public void Apply(IFilter filter)
{
filter.Apply(image);
}
}
public class Caramel
{
public void Init() { }
public void Render(Image image)
{
Console.WriteLine("Applying caramel filter");
}
}
Solution
- CaramelFilter is the adapter and Caramel is the adaptee
- Composition is better than inheritance
public class CaramelFilter : IFilter
{
private Caramel caramel;
public CaramelFilter(Caramel caramel)
{
this.caramel = caramel;
}
public void Apply(Image image)
{
caramel.Init();
caramel.Render(image);
}
}
Example Structure
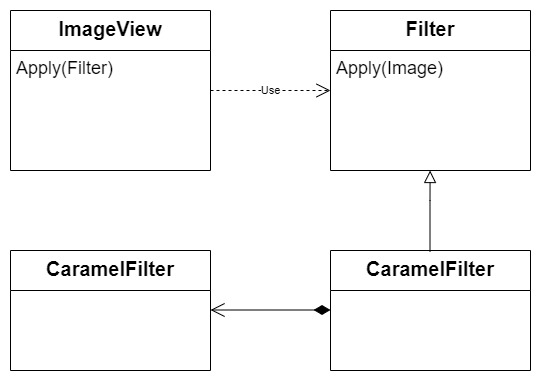
Example Code